Dart extension method in Flutter projects
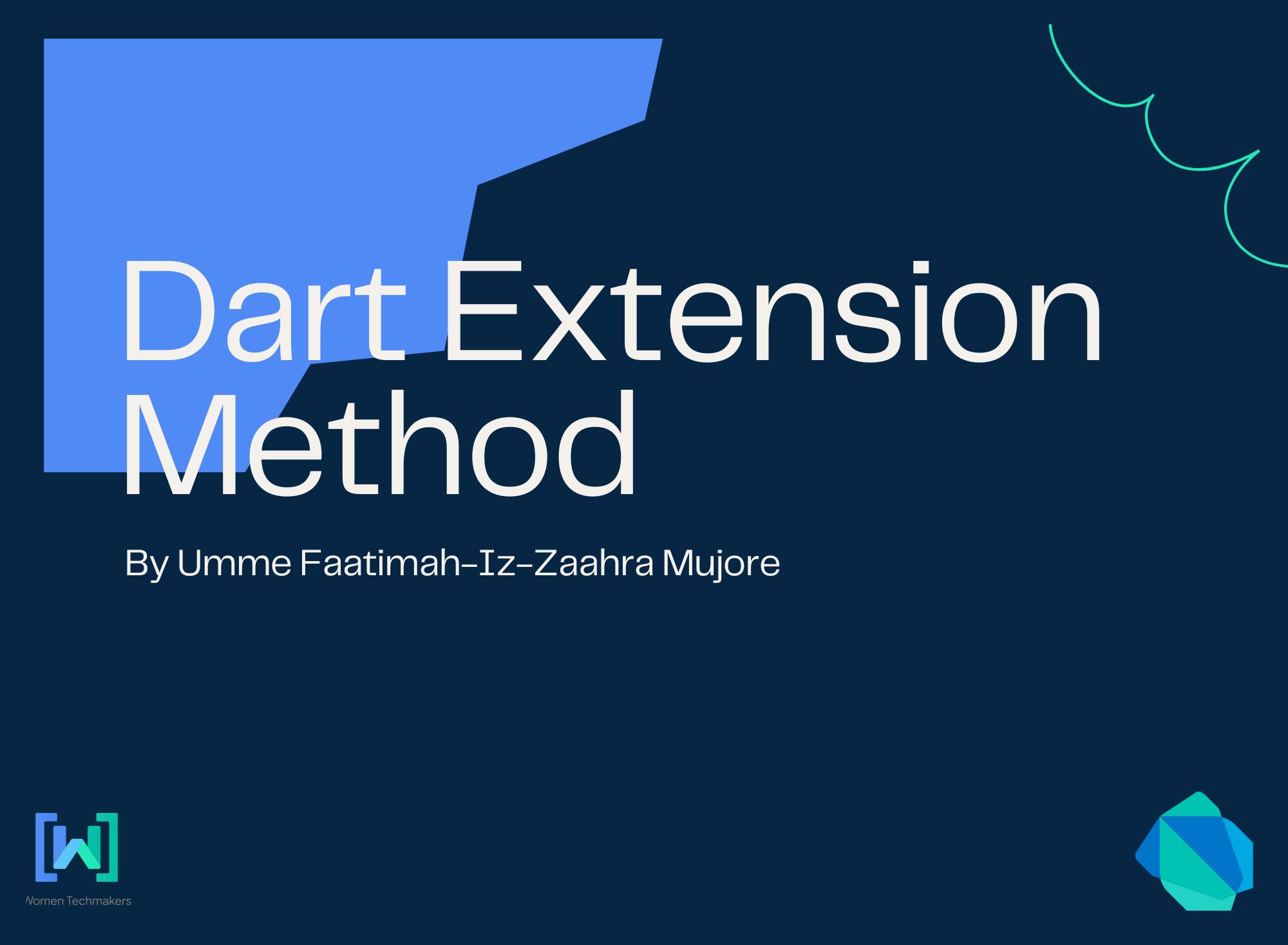
What is the Dart Extension Method?
The Dart Extension Method allows us to add functionality to existing libraries and classes. Extensions can not only define methods but also other members such as getter, setters, and operators.
syntax for Dart Extension Method:
extension <extension name>? on <type> {
(<member definition>)*
}
where ‘extension name’ is the name of the extension and ‘Type’ is the specific class that we want to extend.
How to use the Dart Extension Method in our Flutter project?
Let us review the ‘ExtensionUtils’.
Here, we are extending the Container as this is the class that we want to make changes.
extension ExtensionUtils on Container {
Container addDecoration() {
return Container(
padding: const EdgeInsets.symmetric(
horizontal: 16,
vertical: 8,
),
decoration: const BoxDecoration(color: Colors.blueGrey),
child: this,
);
}
}
And rather than writing code as such:
class NormalWay extends StatelessWidget {
const NormalWay({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
body: Container(
padding: const EdgeInsets.symmetric(
horizontal: 16,
vertical: 8,
),
decoration: const BoxDecoration(color: Colors.blueGrey),
child: const Center(
child: Text(
"Normal way",
style: TextStyle(
color: Colors.white,
fontSize: 24,
),
),
),
),
);
}
}
We will be calling the ‘addDecoration’ method just like we would for an ordinary method and by importing the library in which the method is in.
import 'package:flutter_articles/utils/extension_util.dart';
class FlutterExtensionMethods extends StatelessWidget {
const FlutterExtensionMethods({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
body: Container(
child: const Center(
child: Text(
"Learning extension methods",
style: TextStyle(
color: Colors.white,
fontSize: 24,
),
),
),
).addDecoration(),
);
}
}
Conflicting API
However, an extension member could conflict with an interface or with another extension member if the same extension method is created in 2 different dart files, and one way to solve the conflicting extension would be to use the ‘show’ or ‘hide’ keyword to limit the exposed API.
For example, the ‘AnotherExtensionUtils’ method is the same as the ‘ExtensionUtils’ in a file called the ‘lib/test_utils/test_utils.dart’.
extension AnotherExtensionUtils on Container {
Container addDecoration() {
return Container(
padding: const EdgeInsets.symmetric(
horizontal: 16,
vertical: 8,
),
decoration: const BoxDecoration(color: Colors.red),
child: this,
);
}
}
Therefore, resolving the api would be as follows:
import 'package:flutter_articles/test_utils/test_utils.dart';
import 'package:flutter_articles/utils/extension_util.dart' hide ExtensionUtils;
And that’s it guys. I hope you have a better understanding of how to use the extension method in your Flutter project.
Thank you for reading… ;)
About me
I am Zaahra, a Google Women Techmaker Ambassador who enjoy mentoring people and writing about technical contents that might help people in their developer journey. I also enjoy building stuffs to solve real life problems.
To reach me:
LinkedIn: https://www.linkedin.com/in/faatimah-iz-zaahra-m-0670881a1/
X (previously Twitter): _fz3hra
GitHub: https://github.com/fz3hra
Cheers,
Umme Faatimah-Iz-Zaahra Mujore | Women TechMakers Ambassador | Software Developer